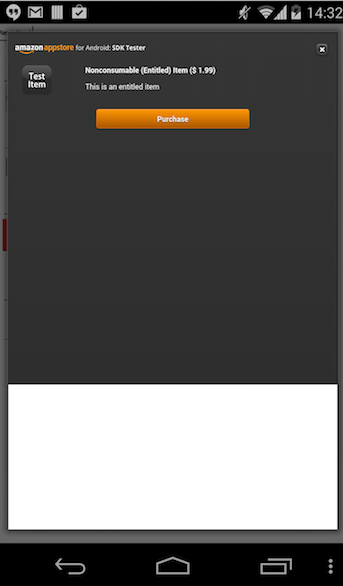
First create three buttons. One button for consumable, one button for non-consumable and one button for subscription. Then add code that allows us to interface with the Amazon Appstore and request the in-app purchases for download.
To our consumable purchase button, add the following code:
on mouseUp
mobileStoreEnablePurchaseUpdates
mobileStoreSetProductType "com.runrev.sampleapp.consumable", "inapp"
mobileStoreMakePurchase "com.runrev.sampleapp.consumable", "1", "This belongs to me"
end mouseUp
Add the following to our non-consumable and subscription purchase buttons:
on mouseUp
mobileStoreEnablePurchaseUpdates
mobileStoreSetProductType "com.runrev.sampleapp.nonconsumable", "inapp"
mobileStoreMakePurchase "com.runrev.sampleapp.nonconsumable", "1", "This belongs to me"
end mouseUp
on mouseUp
mobileStoreEnablePurchaseUpdates
mobileStoreSetProductType "com.runrev.sampleapp.sub.month", "subs"
mobileStoreMakePurchase "com.runrev.sampleapp.sub.month", "1", "This belongs to me"
end mouseUp
Finally, add the following code to the stack script:
on purchaseStateUpdate pPurchaseID, pProductID, pState
switch pState
case "paymentReceived"
answer "payment received!"
offerPurchasedProduct pProductID
mobileStoreConfirmPurchase pProductID
mobileStoreDisablePurchaseUpdates
break
case "error"
answer "Error occured during purchase handling:" & return & return & mobilePurchaseError(pPurchaseID)
mobileStoreDisablePurchaseUpdates
break
case "invalidSKU"
answer "Invalid SKU."
mobileStoreDisablePurchaseUpdates
break
case "alreadyEntitled"
answer "Already Owned."
mobileStoreDisablePurchaseUpdates
break
case "restored"
answer "Restored!"
offerPurchasedProduct pProductID
mobileStoreConfirmPurchase pProductID
mobileStoreDisablePurchaseUpdates
break
case "cancelled"
answer "Purchase Cancelled:" && pProductID
mobileStoreDisablePurchaseUpdates
break
end switch
end purchaseStateUpdate
on offerPurchasedProduct pProductID
if pProductID is "com.runrev.sampleapp.consumable" then
set the cConsumablesCount of this stack to (the cConsumablesCount of this stack + 1)
else if pProductID is "com.runrev.sampleapp.nonconsumable" then
set the cNonConsumablePurchased of this stack to true
else if pProductID is "com.runrev.sampleapp.sub.month" then
set the cSubPurchased of this stack to true
else if pProductID is "com.runrev.sampleapp.sub" then
set the cSubPurchased of this stack to true
end if
end offerPurchasedProduct
The mobileStoreEnablePurchaseUpdates command allows you to monitor the status of each in-app purchase request, using the built-in message, purchaseStateUpdate. This is handled to check the status of the in-app purchase.
The mobileStoreSetProductType command is used to set the type of the in-app purchase. The type can either be "inapp", for consumable and non-consumable items, or "subs" for subscription items. This distinction is not always necessary, since it is only used by the underlying Google API for in-app purchases, because it uses different methods, depending on the item type. However, it is recommended that you always use this command, even for non-Google stores. The mobileStoreSetProductType command is used with the identifier and the type of the in-app purchase we are requesting. These values must match the identifier and the type of an in-app purchase that has been set up in the Amazon Appstore Developer Portal for the app.
The mobileStoreMakePurchase command is then used with three parameters, denoting the in-app purchase identifier we are requesting, the requested quantity of the item and the developer payload. The quantity should always be "1" for non-iOS apps. The developer payload is a string that contains some extra information about the order. This command sends the request and begins the purchasing process. The purchaseStateUpdate message is then generated as the purchasing process takes place. This is sent with three parameters denoting the product identifier, the purchase identifier and the state of the specific purchase that the message is regarding. In the handler, the state of the purchase is checked using the parameter pState. Here we take action if it returns paymentReceived, indicating that payment for the purchase was received, or restored, indicating that the restoration request was successful. In all other cases, we print a message to the screen giving details on what is happening.
In case of an error, mobileStorePurchaseError can be used with the purchase ID to find the details of the error, and then use the mobileStoreDisablePurchaseUpdates command to indicate that the purchase process is complete. This is not essential in all circumstances, but is good practice. mobileStoreDisablePurchaseUpdates is used in cases where the item is already owned by the user, or there is no item with the specified identifier in the store listing, or the user cancels the purchase. In case of payment being received, or the purchase being restored, the app can take action to finalize the purchase or the restoration process. This app permits multiple in-app purchases and we use the pProductID parameter with offerPurchasedProducts to find identify which in-app purchase is being being processed. We can then take appropriate action for the in-app purchase that is being purchased or restored, and use the mobileStoreConfirmPurchase command to confirm to Amazon that payment has been received, finalizing the in-app purchase process on the Amazon Appstore's side.
The bundle identifier that you create the standalone application with, as specified in the standalone application settings, must match the bundle identifier tied to the in-app purchases in Amazon Appstore Developer Portal.